일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- TypeScript
- Javascript
- 삶
- 회고
- 신입 이력서
- 프론트엔드
- 개인 프로젝트
- 백엔드
- CSS
- 신입
- Next.js 13
- 신입 프론트엔드
- aws s3
- 캐플라이어
- 개인프로젝트
- s3 bucket
- 구상
- 대학졸업
- 신입 개발자
- MONGOOSE
- 개발
- react
- 기획
- Next.js
- 계획
- 개발자 이력서
- 이력서
- 공부
- Today
- Total
개발 마라톤
11/4 - easyFetch 함수 추가 본문
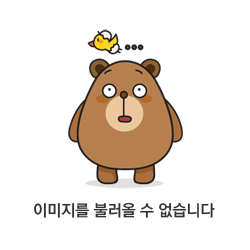
easyFetch 함수 추가
이번 프로젝트에서는 Axios를 채택하지 않았다.
이유는 저번 프로젝트에서 Axios를 채택한 이유가 받아온 데이터를 쉽게 조작할 수 있었기 때문이었는데 Next.js 13 에 내장되어있는 fetch로도 어렵지 않게 구현할 수 있을 것 같아서 Axios를 굳이 채택하지 않았다.
추가적으로 Axios는 구형 브라우저 지원 기능과, response timeout 기능 등의 이점이 더 존재하지만,
지금 구현 중인 프로젝트는 그 정도 규모의 기능을 사용하지는 않을 거 같아서 채택하지 않은 이유도 있다.
Axios와 Fetch의 데이터 처리 방식의 차이점을 살펴보겠다.
// GET 요청
// axios GET
axios.get('/api/users')
.then(response => {
// 응답 데이터 처리
console.log(response.data);
})
.catch(error => {
// 에러 처리
console.error(error);
});
// fetct GET
fetch('/api/users')
.then(response => response.json())
.then(data => {
// 응답 데이터 처리
console.log(data);
})
.catch(error => {
// 에러 처리
console.error(error);
});
출처 : fetch 보다 axios를 쓰는 이유 (tistory.com)
Axios의 경우 받아온 response를 데이터에 접근할 수 있도록 자동적으로 처리해준다.
즉, response.json() 이 불필요하다.
// POST 요청
// axios POST
axios.post('/api/users', { name: 'John', age: 25 })
.then(response => {
// 응답 데이터 처리
console.log(response.data);
})
.catch(error => {
// 에러 처리
console.error(error);
});
// fetch POST
fetch('/api/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name: 'John', age: 25 })
})
.then(response => response.json())
.then(data => {
// 응답 데이터 처리
console.log(data);
})
.catch(error => {
// 에러 처리
console.error(error);
});
POST 요청의 경우도 살펴보자.
Axios의 경우 headers를 번거롭게 설정해주지 않아도된다.
즉, headers가 기본적으로 설정되어있다.
fetch가 axios에 비해 단점이라고 생각되는 요소를 정리해보자.
- response.json() 가 필요하다.
- 번거로운 headers 설정이 필요하다.
즉, 이 두 가지 단점을 해결할 수 있다면 fetch도 충분히 쓸만한 함수라고 생각한다 !
그래서 나는 easyFetch라는 함수를 작성하기로 했고, 아래는 변화된 예시이다.
export async function postLogin(formData: FormData): Promise<{message : string; status : number}> {
const response = await fetch(`${URL}${API_ROUTES.SESSION}`, {
...POST_FORM,
body: formData,
});
const data = await response.json();
return { ...data, status: response.status };
}
export async function postLogin(
formData: FormData
): Promise<TypeResponse<object>> {
const data = await post(URL, API_ROUTES.SESSION, formData);
return data;
}
같은 postLogin 함수임에도 더 직관적으로 변했음을 확인할 수 있다.
easyFetch는 fetch와 비교해서 다음과 같은 처리를 가미했다.
- response.json()을 내부에서 수행한다.
- header 객체로 간편하게 GET, POST 등의 메소드 및 헤더를 설정한다.
- data와 status를 한 번에 반환한다.
코드는 아래와 같다.
// easyFetch.ts
// easyFetch header
const header = {
GET: {
method: 'GET',
headers: {
'Content-Type': 'application/json',
},
},
POST: {
method: 'POST',
headers: {
// 'Content-Type': 'multipart/form-data'
// 해당 값은 encType에 의해 자동으로 지정될 것임.
},
},
};
// 커스텀 fetch 함수
type TypeHeader = {
method: string;
headers: HeadersInit;
};
export const easyFetch = async (
url: string,
route: string,
header: TypeHeader,
params?: string,
options?: object
) => {
const response = await fetch(`${url}${route}?${params}`, {
...header,
...options,
});
const data = await response.json();
return { data: data, status: response.status };
};
위의 easyFetch를 활용하여 더 사용하기 쉬운 get, post 함수도 작성할 수 있었다.
// 각 method에 따른 함수
export const get = async (
url: string,
route: string,
params?: string,
options?: object
) => {
return easyFetch(url, route, header.GET, params, options);
};
export const post = async (
url: string,
route: string,
data: any,
params?: string,
options?: object
) => {
return easyFetch(url, route, header.POST, params, { ...options, body: data });
};
'--- Project --- > CharFlyer : 캐플라이어' 카테고리의 다른 글
[개발] Next.js 13 JWT 재발급 (작성중) (0) | 2023.11.10 |
---|---|
[개발] Next.js 13 JWT 발급 (1) | 2023.11.06 |
10/31 - Server Component / Client Component 분할 (0) | 2023.10.31 |
10/25 - Next.js 13 깔끔한 폴더 구조 (0) | 2023.10.25 |
10/24 - RESTful API 고민하기 (0) | 2023.10.24 |